Component Data Services
Autobind
var config:config = { dataSources: { SalesOrder: { url: "sandbox/sales/orders/{salesOrderId}", autoBind: true } } }
If autoBind is set to true the component will automatically execute a GET request on the specified API resource when the application is loaded. Autobind will also automatically execute a PUT request if the application user has changed the value of any input fields. This means that in simple use cases it is not necessary to write any javascript/typescript in the viewModel when the component should only display and update data.
vm.afterDataAutoLoaded = function () { if (vm.salesOrder) { //do something with vm.salesOrder } }
Tip
If you need more control over the dataset there is a callback function available named: vm.afterDataAutoLoaded. This function is executed when the API GET response is returned to the client. This makes it possible to manipulate the data before it's displayed to the user.
vm.afterDataAutoLoaded is executed everytime an autoBind resolves from an GET or PUT request. So it can be important to check if it is the dataset that you are currently interested in that are being returned.
GET and PUT request on autoBind resources including vm.afterDataAutoLoaded are executed in this cases:
Initial load of the component
On parameters change
User triggered save (Ctrl + s)
Programatically update of autoBindings (ds.updateAutoBindings)
Note
autoBind can also be set to the value "GET". In this case the framework is only executing GET requestes, and not PUT requests. Meaning the data will only be displayed to the end user, and nothing will be saved even if the value of input fields are beeing changed in the UI.
The template generater rb template will create form elements using the autoBind property.
CRUD operations
Read
ds.executeQuery("sandbox/sales/orders/{salesOrderId}").then(function(data) { vm.dataToDisplay = data.salesOrders; });
Tip
If you need more control over when to execute a GET request you should use this function. All API requests against Rambase.net are asynchron so you are only guaranteed that the dataset is available in the then callback.
Update
var model = {"salesOrder": vm.salesOrder}; ds.update("sandbox/sales/orders/{salesOrderId}", model).then(function(data) { console.log("data saved"); });
Tip
If you need more control over when to execute a PUT request you should use this function. The update model must be a json object. Only fields that are dirty (changed) will be put to the API.
Create
var model = {"salesOrder": vm.salesOrder}; var model = { salesOrder: { customer: { customerId: 100008 }, currency: "NOK" } } ds.insert("sandbox/sales/orders", model).then(function(data) { console.log("data saved"); });
Use this function to POST data to the RamBase API.
Delete
ds.delete("sandbox/sales/orders/{salesOrderId}").then(function(data) { console.log("item deleted"); });
Use this function to DELETE data.
Metadata
When you execute a GET request the framework will automatically execute an extra request for GET and PUT metadata.
GET metadata
GET metadata provides the description text on rb-input labels:
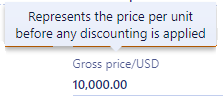
For list resources it will in addition provide information about filterable and sortable fields:
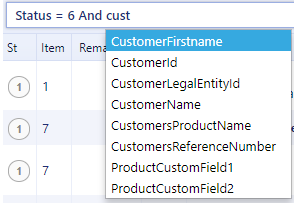
PUT metadata
PUT metadata is used to restrict access to edit and inspect data by applying permissions and access-rules to the API fields.
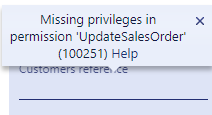
By using the rb-input element you will automatically get metadata for that specific field. If this specific field does not have metadata you can apply metadata from antoher field coming from the same resource by using the attribute metadata-model.If you for some reason are not using rb-input you can apply metadata on a regular input field by using the attribute rb-validate.
Exclude metadata
If you don't want to use metadata on an rb-input element you can do that by specifying the attribute no-validate.
If you don't want to use metadata on an regular input element you can do that by specifying the attribute rb-no-validate.
If you don't want to recieve any metadata for the GET request you must use this syntax:
var query = new ds.Query().from("sandbox/sales/orders/{salesOrderId}"); query.excludeMetadata(); query.excludePutMetadata(); ds.executeQuery(query).then(function(data) { vm.salesOrder = data.salesOrder; });
Communicating between components
Parametermapping
RamBase components can change and listen to parameter changes. Parameters must be defined in the config file. When a component is added to an application from appeditor, the component parameters needs to be mapped to the application parameters.
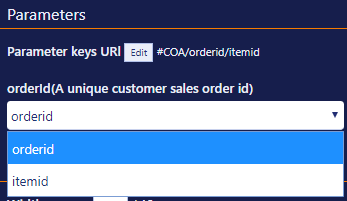
A component can inspect the current parameter values by executing this function:
ds.parameters(); //{orderId: "107580", orderItemId: "1"}
A component can change url parameters by executing this function:
ds.changeUrlParameter("orderId", 100000);
A component can listen to url parameter changes by using this function:
vm.onParametersChanged = function(newParameters, previousParameters) { console.log(newParameters.orderId); }
Parameters can also be passed to RamBase lookup components, like a popup or popover component. Ref. popup under Component UI Elements.
Listen to API requests
ds.registerListeners(vm, "put", "sales/orders/{salesOrderId}, function (verb, uri, obj, promise) { promise.then(function (data) { }); }
If you need to update something in component B after component A has executed an API request, this can be achived by using the registerListeners method. Mandatory parameters are the viewModel, the HTTP verb and the url to listen to. The method also provides a callback promise if you want to execute your code logic after the request has resolved.
Navigation
Used for navigating to RamBase applications
ds.rbNavigate("COA");
HTML loaded
Lifecycle event. Triggered when the component HTML has loaded.
vm.onHTMLLoaded = function() { }
Save changes
In RamBase most of the applications use autosave. Meaning save happens without direct user interaction, instead changes are saved when form elements are being blurred or when the location parameter changes. If you are using autobind in the config file, or if you have implemented a save logic on blurring input fields etc. you don't have to worry about the vm.saveChanges method.
vm.saveChanges = function() { vm.myOrder.customersReference = "test2"; ds.update("sales/orders/107878", {salesOrder:vm.myOrder}); }
If that's not the case then you can use the saveChanges event to implement your custom save logic. vm.saveChanges are triggered before any location parameter changes, and when the user press ctrl+s. If you PUT on the same model object that you got form ds.executeQuery the framework will handle the dirty checking, if not then you probably want to check if your model has changed before executing the PUT request. If you inspect the vm.myOrder object after receiving the data from the GET request, you will see an $originalCopy object which contains all the original input values for the model. It is this object which the framework use for dirty checking the model.
ds.executeQuery("sales/orders/107878").then(function(data) { vm.myOrder = data.salesOrder; console.log(vm.myOrder.$originalCopy); });
Get / save application state
savedApplicationState is used for saving state in the application which can be fetched from getApplicationState if the user returns to the application after a navigation event. The application state is saved for the current application in the current history point. Use JSON.stringify(value) and JSON.parse(value) if you need to save a js object.
ds.saveApplicationState(key:string, value:string); ds.getApplicationState(key:string);
Clear model
Used for recursively deleting object properties.
ds.clearModel(vm.myObject);
appmatch, appNumber and folderPath
folderPath returns the relative path to the component folder. vm.appMatch returns the component name and vm.appNumber return the unique component number.
<img src="{{vm.folderPath}}/images/capture.png" />
Trigger api operation
Operations are used when the standard http GET, PUT, POST, DELETE are not enough. More exact, instead of updating or reading information about an object, we perform an operation on the object. The first parameter is the url of the api resource the api operation is attached to. The second parameter is the number of the api operation, the third is the input object, and the last is whether the application should be reloaded after the api operation has resolved.
ds.triggerApiOperation("sales/orders/{orderId}", 100042, jsonData, true).then( function(data) { toastr.success("Confirmed deliverydate successfully updated."); });
User service
The userservice returns information about the active user of the system or other information from the current session. Remember to inject the rbUserService as an angular service.
rbUserService.getPid(); rbUserService.getDb(); rbUserService.getTarget(); rbUserService.getLanguage();
rbGlobals
rbGlobals.isMobileView(); //Checks if the screen size is maximum 500px wide rbGlobals.isTabletOrMobileView(); //Checks if the screen size is maximum 1024px wide rbGlobals.historyBack(); //Navigates to the previous application in the history list